TL;DR: Every SSH Key on Tumblr.
IF MY DEMANDS ARE NOT MET I WILL GENERATE AND PUBLISH AN SSH KEY EVERY 3 HOURS, SLOWLY REDUCING THE ENTROPY OF THE AVAILABLE SSH KEYSPACE AND REVEALING EVERYONE'S PRIVATE KEYS.
— Comrade Snowy (@EmperorSnowy) May 15, 2021
The above tweet, from my panther friend, struck a chord with me. So working with them I decided to throw together a quick bot that would generate and publish SSH keys on a regular basis.
Concept
When you generate an SSH key (focusing on 2048 bit RSA keys here), you pick two prime numbers that are within a range of bits long. This does mean there is a finite space in which these keys can exist, although likely one that is very large. In fact, based on some math online we can estimate the key space at approximately (sqroot(2^2048)/ln(sqroot(2^2048)))^2.
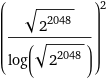
Throwing it into Wolfram Alpha we can see this gives us approximately 6.414 * 10^610 combinations of keys.
From a practical standpoint, generating one every three hours we’re likely not even going to collide with an existing, in-use key before the heat death of the universe. Likely we could not even store them all, even if every electron on Earth was dedicated to holding keys we couldn’t publish them all by a looooong shot.
But this isn’t about practicality. This is just about the concept of keys, of finite spaces in which we work, and a joke about threatening to reveal all secrets through the process of brute force and elimination.
I mean, we’re also only tackling 2048 bit RSA, nevermind 4096 bit RSA or Ed25519.
Implementation
I realised that we couldn’t do this through Twitter, the length of keys in the popular PEM format exceed Tweet allowances, and doing them as images wouldn’t allow for enough accessibility text. So I decided to set up a Tumblr for this purpose: Every SSH Key after discovering their API would be easy enough to integrate with.
To generate the keys, I take advantage of the Node.js inbuilt crypto
API which allows us to create keys quickly and then shunt them through as text posts to the Tumblr. This is done every three hours - until Snowy’s demands are met.
For anyone interested:
const crypto = require('crypto');
const tumblr = require('tumblr.js');
const client = tumblr.createClient({
consumer_key: process.env.TUMBLR_CONSUMER_KEY,
consumer_secret: process.env.TUMBLR_CONSUMER_SECRET,
token: process.env.TUMBLR_TOKEN,
token_secret: process.env.TUMBLR_TOKEN_SECRET
});
generateAndPostSSHKey()
setInterval(generateAndPostSSHKey, 10800000);
function generateAndPostSSHKey() {
crypto.generateKeyPair('rsa', {
modulusLength: 2048,
publicKeyEncoding: {
type: 'pkcs1',
format: 'pem'
},
privateKeyEncoding: {
type: 'pkcs1',
format: 'pem'
}
}, (err, publicKey, privateKey) => {
if (err) {
console.log(err);
return;
}
const body = publicKey + '\n\n' + privateKey;
client.createTextPost(process.env.TUMBLR_BLOG_NAME, {
body: body
}, (err) => {
if (err) {
console.log(err);
}
});
})
}
PS: You may notice that we don’t check if an SSH key has already been generated before! There’s no search API on Tumblr that would let us check if we’ve posted one before, and we’re not paying for the amount of disk space required (see above assumptions) and we’re pretty sure the universe will have faded by the time we’re even likely to hit a collision (see above assumptions).